Throwable and Error in Java
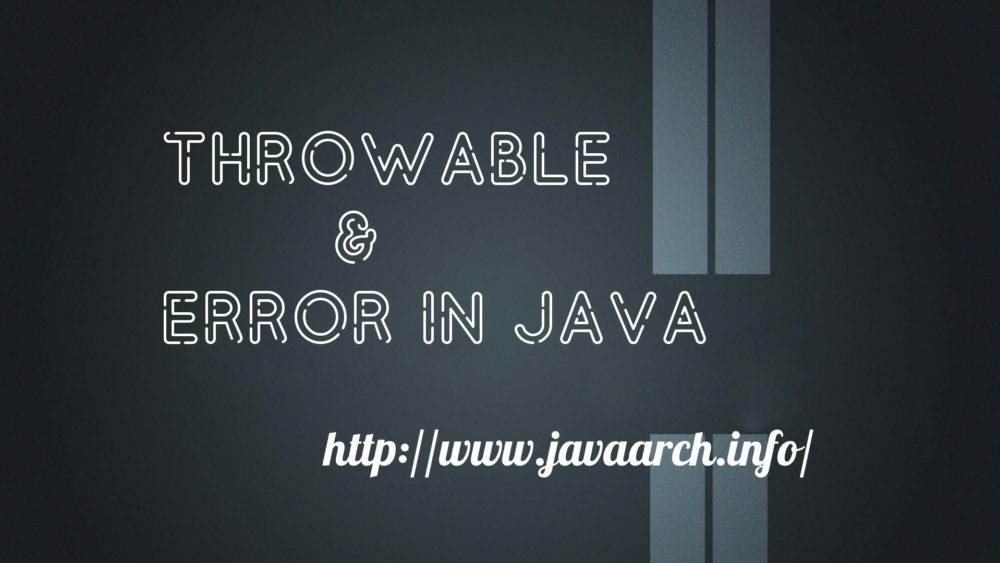
In last discussion, we discussed on Exceptions and handling the exception. Now we would discuss one level up in the exception hierarchy Throwable and Error. Throwable class provides basic implementation for handling serious issue or recoverable issue in the code. All the Throwable and child of Throwable class objects can be thrown and handled in catch block.
Error and Exception are subclasses(child) of Throwable. Each subclasses designed for specific purpose.
Error : An Error is thrown to indicate a serious problem in the application. Error catch block mostly to send alert to user. Most of the Error could not be recovered or continue the process such as stackOverflowError, OutOfMemoryError, NoClassDefFoundError.
Errors can be thrown and handled in catch block.
package com.javaarch;
public class ErrorTest {
public static void main(String[] args) {
try {
// Code thrown out of memory error
}catch(OutOfMemoryError e) { //Error
System.out.println(“Send critical email alert”);
// Send an email and alert notification to user.
// Stop the process and it can’t be continued.
}
}
}
Exception : Exceptions are used to handle logic issue or undesirable behaviour from external system. For example, only digits allowed in age field. If user enters any special character values in age field, it should be handled and prompt again for user inputs. Exception examples are server could not be reached due to a network problem. Not able to find an file for file processing.
Handling of Exception can be classified as “Checked Exceptions” and “Unchecked Exception”. Subclasses of “RuntimeException” are unchecked exception. We will discuss more on checked exception and unchecked exception in next post.
Errors are errors, not an exceptions.
On final note, Error can be thrown in case of serious issue such as JVM issue, memory issue or security hack in the code. Most of the time error catch block handle the error by sending alert mail or notification to user and stop the execution. Errors are different from exception. Happy Coding:)