Checked Exception and UnChecked Exception
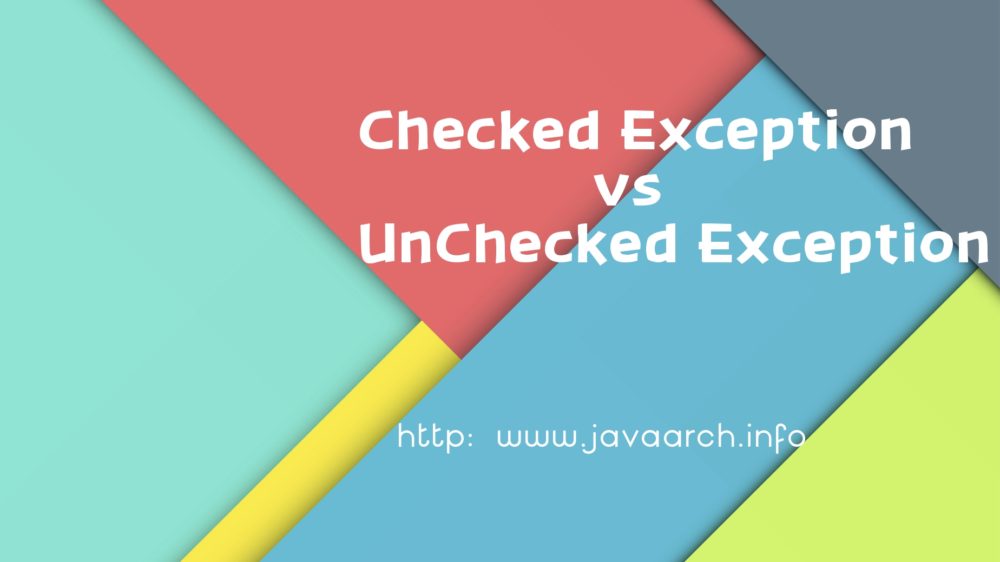
Java provides framework to catch the exception and handle the exception by try…catch block. Java exceptions are grouped into two major categories checked exception and unchecked exception. Checked exception are caused by undesirable or unexpected condition. It can be recoverable by handling the issue in catch block such as one of the server port in not accessible, File not found in the folder. Unchecked exception are caused by logic issues or problematic issues in the code such as Null pointer exception, Array index out of bound exception.
Exception by Undesirable Condition – Checked Exception.
Exception by logic and programetaic error – Unchecked Exception.
Both checked exception and unchecked exception can be handled in try…..catch block. But programmer have decide based on the logic.
Checked Exception:
Checked exception are exception caused by undesirable condition such as server not able to connect or file not found error. These exception can be handled and recovered by trying to connect to another server port or searching file in different folder. Checked exception are assumed that it would be thrown in undesirable condition and can be recoverable. So jvm forces developer to handle the checked exception. Otherwise it would throw compile time error.
String host = “jdbc:derby://localhost:1527/Employees”;
String uName = “root”;
String uPass= “password”;
Connection con;
try {
con = DriverManager.getConnection( host, username, password );
String host = “jdbc:derby://localhost:1527/Employees”;
String uName = “root”;
String uPass= “password”;
}
catch ( SQLException err ) { // Checked exception and it is recoverable by connecting to server 2
System.out.println( “Error in creating connection to localhost…Reconnecting to server 2”. );
String host = “jdbc:derby://server2:1527/Employees”;
con = DriverManager.getConnection( host, username, password );
}
Checked exception are
- thrown by Undesirable condition such as server failure or file not found.
- Can be recovered by handling the exception in catch block and retry.
- JVM forces developer to handle the exception to continue the execution.
- Handle the exception using try-catch block or it should propogate the exception using throws keyword, otherwise the program will give a compilation error.
Unchecked Exception:
All the sub classes of RuntimeException class are considered as unchecked exception. Unchecked exception are caused by logic issues or problematic issues in the code such as null pointer exception, arithmetic issues, divide by zero issues. Even unchecked exception can be handled and continue the execution. But JVM assumes unchecked exception are logic error and can’t continue the execution. So JVM does not force to handle the unchecked exceptions.
Unchecked Exception,
- thrown by logic issues or problematic issues in the code.
- Can be handled in catch block.
- JVM assumes unchecked exception can’t be recoverable, So it never force to handle the exception in catch block.
- requires code changes to fix the issue. So can’t continue the execution without modifying the code.
try{
int arr[] ={1,2,3,4,5};
System.out.println(arr[7]);
}
catch(ArrayIndexOutOfBoundsException e){ // Unchecked exception and could not continue the code without modifying the code.
System.out.println(“The specified index does not exist ” +”in array. Please correct the error.”);
}
Note : JVM assumes the unchecked exception can’t be recoverable and continue the execution. So it doesn’t force to handle the unchecked exception. This same logic applies for Throwable and Error as well. No specific catch block is forced for Throwable and Error.
JVM does not force to handle the Throwable and Error like unchecked exceptions.
On final note, checked exception and unchecked exception are not same. Both exception to notify different type of exceptions in the code. Few programmers says checked exception are compile type exceptions and unchecked exceptions are run time exceptions. It is totally wrong. All the exception are run time exception including Throwable, Error, Checked exception and unchecked exceptions. Happy Exception Handling.:)