finally block in Java – Success or failure. Guarantee to be executed
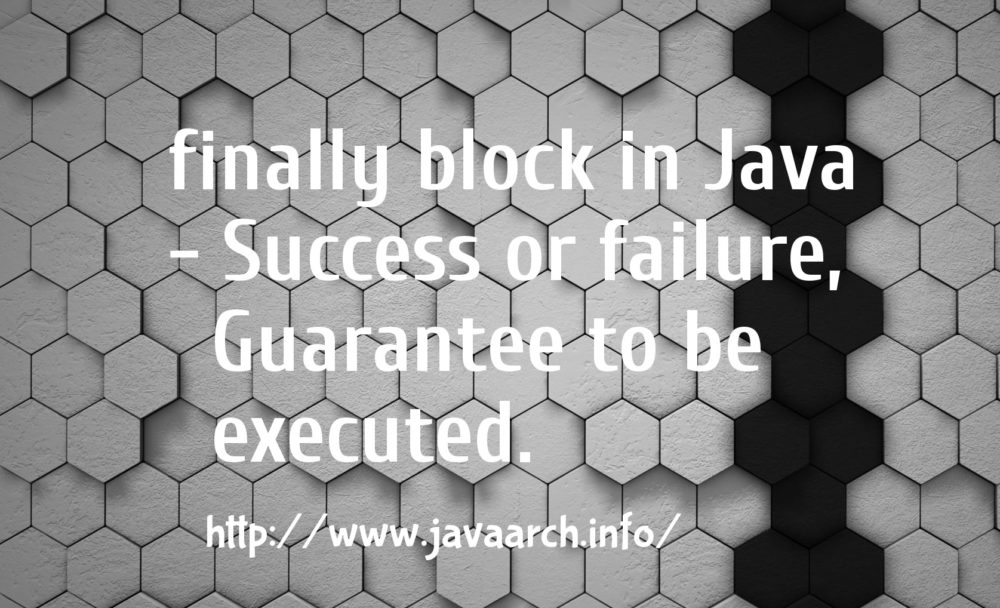
In last post, we discussed on exception handling, checked exception. try…catch block helps to handle the exception. Let us discuss DRY principle before discuss on finally block. The DRY(Don’t Repeat Yourself) is a principle of software development aimed to avoid repetition of same code in multiple places.. The DRY principle is stated as, “Every piece of knowledge or logic must have a single, unambiguous representation within a system.”. Let us take below example,
package info.javaarch;
import java.io.*;
public class TestFinally {
public static void main(String args[]) throws IOException {
FileInputStream in = null;
FileOutputStream out = null;
try {
in = new FileInputStream(“studentlist.txt”);
out = new FileOutputStream(“studentnames.txt”);
int c;
while ((c = in.read()) != -1) {
out.write(c);
}
in.close(); // Repeat 1
out.close();
} catch (FileNotFoundException e) {
System.out.println(“ERROR:Not able to find the files.”);
in.close(); // Repeat 2 – Against DRY principle
out.close();
}
}
}
The input streams have to be closed in try as well as in catch block. Same code repeated twice and it is against DRY principle. Most of the real time scenario, some block of the code should be executed regardless of success or failure scenario. Java enables the always run by “finally” block.
A finally block is always executed ,it doesn’t matter if the try block throws an exception, whether or not the exception is caught, or if it executes a return statement. “finally” block would be used to release the JDBC connections, closing the stream and freeing up the resources. “finally” block would be executed before return the method result if any return value.
finally with try…catch block..
“finally” block would be written after all the catch block if catch block exist for the try.
try {
in = new FileInputStream(“studentlist.txt”);
out = new FileOutputStream(“studentnames.txt”);
…….
} catch (FileNotFoundException e) {
System.out.println(“ERROR:Not able to find the files.”)
}finally {
in.close(); // code at only one block, never repeated.
out.close();
}
“finally” without catch block:
It can exist without catch block. But try block should have atleast catch or finally.
try {
in = new FileInputStream(“studentlist.txt”);
out = new FileOutputStream(“studentnames.txt”);
…….
}finally {
in.close(); // code at only one block, never repeated.
out.close();
}
Use finally to release the resource or compulsory code to be executed for the workflow. It greatly helps to avoid unnecessary repetition of the code. Use finally to adhere DRY principle in your code. Happy Coding 🙂