Concurrent simplified using one line of code in Java ExecutorService and ThreadPoolExecutor
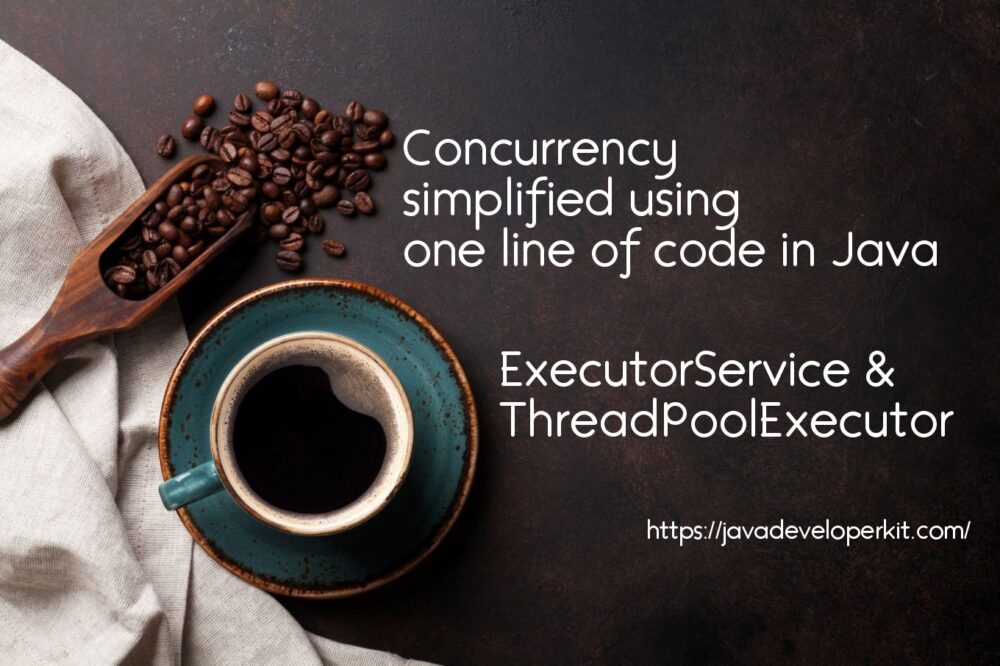
The Java ExecutorService interface, java.util.concurrent.ExecutorService, represents an asynchronous execution mechanism which is capable of executing tasks concurrently in the background. In this post, We would discuss list of interfaces to run task in parallel in Java using Concurrency utilities.
public interface Executor – An Executor that provides methods to manage termination and methods that can produce a Future for tracking progress of one or more asynchronous tasks.
public interface ExecutorService extends Executor – An ExecutorService can be shut down, which will cause it to reject new tasks. Two different methods are provided for shutting down an ExecutorService
The Executors gives factory methods Executors.newCachedThreadPool() (unbounded thread pool, with automatic thread reclamation), Executors.newFixedThreadPool(int) (fixed size thread pool) and Executors.newSingleThreadExecutor() (single background thread), that preconfigure settings for the most common usage scenarios.
ExecutorService executorService = Executors.newFixedThreadPool(10);
executorService.execute(new Runnable() {
public void run() {
System.out.println(“Asynchronous task”);
}
});
Scheduled Executor Service :
To schedule the task at specified interval and fixed delay, we can use ScheduledExecutorService.
public interface ScheduledExecutorService extends ExecutorService – The scheduleAtFixedRate and scheduleWithFixedDelay methods create and execute tasks that run periodically until cancelled.
ScheduledExecutorService scheduledExecutorService = Executors.newScheduledThreadPool(1);
Runnable task = () -> {
System.out.println(“Executing Task At ” + System.nanoTime());
};
System.out.println(“scheduling task to be executed every 2 seconds with an initial delay of 0 seconds”);
scheduledExecutorService.scheduleAtFixedRate(task, 0,2, TimeUnit.SECONDS);
ThreadPoolExecutor :
In case to control the number of tasks in the waiting queue and throw exeception in case more number of tasks received in the blocking queue, we have to use ThreadPoolExecutor. It extends AbstractExecutorService.
public class ThreadPoolExecutor extends AbstractExecutorService
ThreadPoolExecutor(int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue<Runnable> workQueue)
Creates a new ThreadPoolExecutor with the given initial parameters and default thread factory and rejected execution handler.
corePoolSize – the number of threads to keep in the pool, even if they are idle, unless allowCoreThreadTimeOut is set
maximumPoolSize – the maximum number of threads to allow in the pool
keepAliveTime – when the number of threads is greater than the core, this is the maximum time that excess idle threads will wait for new tasks before terminating.
unit – the time unit for the keepAliveTime argument
workQueue – the queue to use for holding tasks before they are executed. This queue will hold only the Runnable tasks submitted by the execute method.
public ThreadPoolExecutor(int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue<Runnable> workQueue, RejectedExecutionHandler handler)
corePoolSize – the number of threads to keep in the pool, even if they are idle, unless allowCoreThreadTimeOut is set
maximumPoolSize – the maximum number of threads to allow in the pool
keepAliveTime – when the number of threads is greater than the core, this is the maximum time that excess idle threads will wait for new tasks before terminating.
unit – the time unit for the keepAliveTime argument
workQueue – the queue to use for holding tasks before they are executed. This queue will hold only the Runnable tasks submitted by the execute method.
handler – the handler to use when execution is blocked because the thread bounds and queue capacities are reached
ExecutorService executorService = new ThreadPoolExecutor(10, 100, 5L, TimeUnit.MILLISECONDS, new LinkedBlockingQueue<Runnable>());
Basic example to start executorservice : https://jenkov.com/tutorials/java-util-concurrent/executorservice.html
Fixed Thread Pool Example : https://howtodoinjava.com/java/multi-threading/executor-service-example/
Customized Thread Pool Executor : https://www.callicoder.com/java-executor-service-and-thread-pool-tutorial/
Thread Pool Executor with Rejected Exception example : https://www.digitalocean.com/community/tutorials/threadpoolexecutor-java-thread-pool-example-executorservice
Thead pool executor with Listener : https://www.baeldung.com/thread-pool-java-and-guava