Handle the unexpected issue in your code – Exception Handling in Java
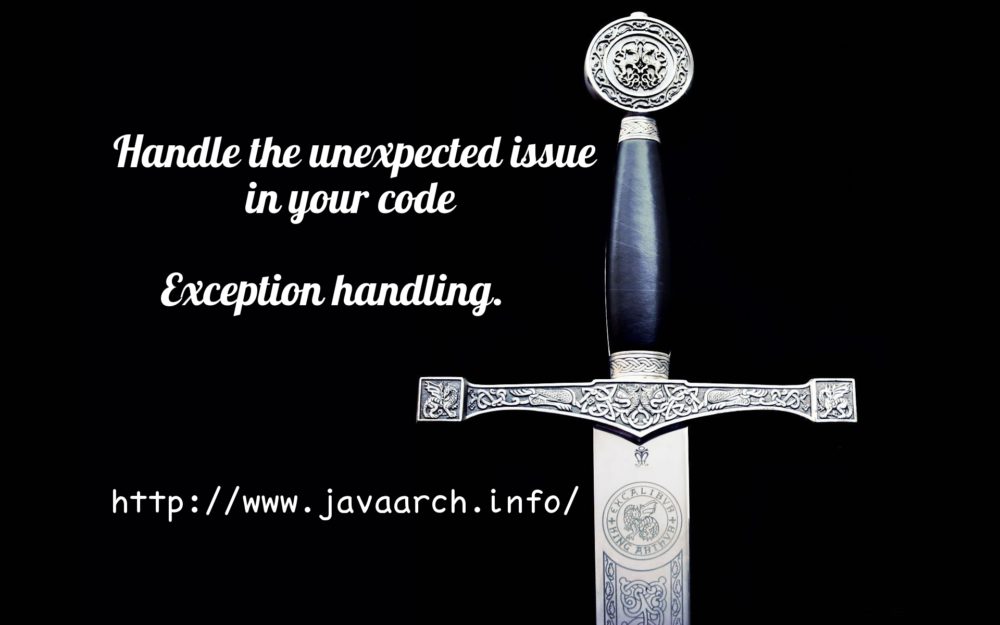
In software industry, 80% of the effort spend on debugging and resolving the issues in the application. So identifying the issues, finding the problematic code block and handling the issues are important. It would greatly reduce the programmer’s effort to debug and fix the issue. Java provides elegant way to identify and handle the issue by Exception handling in java. Let us discuss basics of exception handling in this post.
Exception handling powered 3 basic blocks:
1). Application logic which would throw exception in case of issue.
2). Actual Exception. Exception name, Exception type.
3). Exception handling code.
try{
……..
…….. // 1). Application logic which would throw exception in case of issue.
} catch(IOException e){ // 2). Actual Exception. Exception name, Exception type.
…………. // 3). Exception handling code.
}
try catch :
In exception occurs in try block, the flow immediately jumps into exception handling code. The next line of the code will not execute in the try block. Properly designed exception handling would help us to identify and resolve the issue. Each block should be written logically and handle the exception. Writing single try and catch block may not help us to identify and handle the issue. Look at below snippet. It handles all the exception in one block. It is unnecessary and tedious to deduct the type of exception.
try{
…….
}catch(Exception e){
………….
}
Instead of handling all the exception in generic catch, handle only the expected exceptions. Handle each exceptions logically by different catch block. It would provide different flow of code based on exception. The below exception handling code gives 4 different flows.
1). Proper execution flow
2). Failed exception with arithmetic exception.
3). Retry by getting new set of inputs and calculate age with InputMismatchException.
4). Calculate the age by algorithm 2 if algorithm 1 fails with DateTimeParseException.
public static void calculateAge(int day, int month, int year) { // Implementation 1 with multiple catch block.
try {
//calculate age by day,month and year by algorithm 1
}catch(ArithmeticException e) {
System.out.println(“failed execution.Terminating….”);
}catch(InputMismatchException e) {
System.out.println(“Inputs mismatch. Kindly reenter the value.Retrying…”);
//Get the values again and retry calculate age by day,month and year
}catch(DateTimeParseException e) {
System.out.println(“Logic failed in algorithm 1. Calculating the age by algorithm 2 with different date time format.”);
//Use algorithm 2 with different date time format. calculate age by day,month and year by Algorithm 2.
}
}
public static void calculateAge(int day, int month, int year) { // Implementation 2 with single catch block
try {
//calculate age by day,month and year
}catch(Exception e) { // Generic handling of exception
System.out.println(“failed execution.Terminating….”);
}
}
Log the exception information in the catch block. Empty catch block would hide the actual issue and make issue identification job more complex. Compare the power of exception handling by above 2 different implementations.First implementation is more powerful than generic implementation. Proper exception handling saves your 90% of debugging time.
Proper exception handling saves your 90% of debugging time.
List all the exception prone code and handle the exception. Write exception handling for all the expected exception in the try block. Never leave the catch block without logger message. Worst case, print the actual exception in the catch block if no exception handling code logic. In simple terms, exception handling is like sword. If we handle it properly, it would give you extra power to do the job. Otherwise it would hurt us heavily. Same analogy applicable with exception handling as well.
try {
…….
}catch(IOException}{
// No statement in catch block and no logger/print message. // Worst exception handling.
}
Never leave catch block without any logger message. Always log or print the actual exception for debugging.
Here we discussed basic of exception handling and how to write simple exception handling. We would discuss further on exception handling with finally, checked exception and unchecked exception in upcoming posts. Stay tuned. Happy Exception Handling 🙂