Modifiable Strings by StringBuilder & StringBuffer
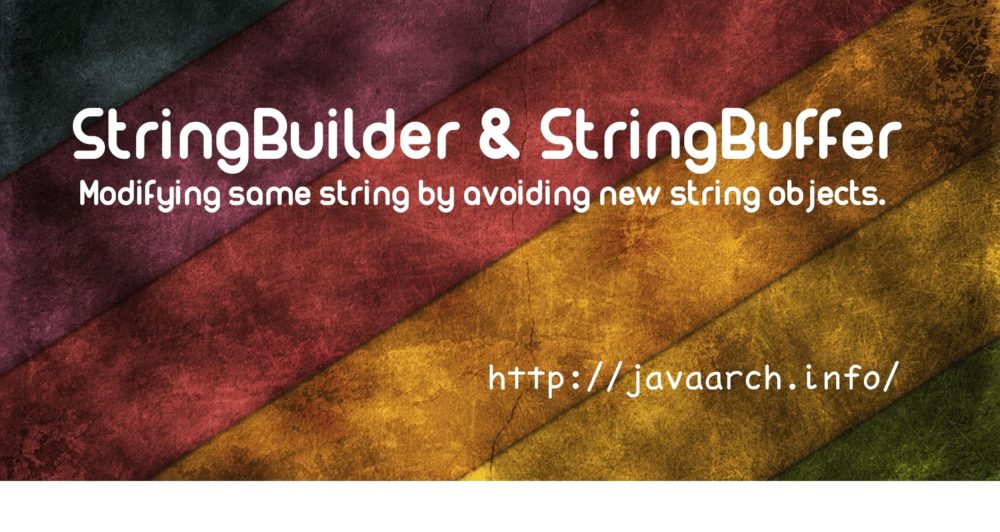
In previous blog, we discussed on String and string constant pool. It highlighted the issue in String manipulation and how it fill the pool memory. We not recommended to use String for temporary string statement formation, logger statement, updatable string values, large file I/O stream of input processing. We can process these operation using String , but it would create lot of abandoned strings in string constant pool and inefficient use of java memory. Even with gigabytes of RAM, it’s not a good idea to waste precious memory on discarded String pool objects. Instead of String, there is better alternative to use for these use cases. StringBuilder & StringBuffer.
StringBuffer:
Let us explore the StringBuffer API’s. StringBuffer is introduced to handle modifiable string with guarantee of synchronization in multithreaded environment. It gives powerful API to manipulate the Strings.
StringBuffer() : constructor creates an empty string buffer
StringBuffer(String str) : constructor creates a string buffer with the specified string.
StringBuffer sb = new StringBuffer(); // Creates new StringBuffer object.
sb.append(“Java”); // Appends the String value in StringBuffer.
sb.append(1.8); // append() can be used to append string,double,float,long,int,char,boolean or another stringbuffer object.
sb.charAt(index) // Returns character at index position.
sb.delete(int start, int end) // Removes the characters in a substring of this sequence.
sb.deleteCharAt(int index) // Removes the char at the specified position in this sequence.
sb.indexOf(String str) // Returns the index within this string of the first occurrence of the specified substring.
sb.insert(int offset, value) // Inserts the string representation of the value(type of double,boolean,char,float,int,long and String) argument into this sequence.
sb.lastIndexOf(String str) // Returns the index within this string of the rightmost occurrence of the specified substring.
sb.length() // Returns the length (character count).
sb.replace(int start, int end, String str) // Replaces the characters in a substring of this sequence with characters in the specified String.
sb.reverse() // Causes this character sequence to be replaced by the reverse of the sequence.
sn.substring(int start) // Returns a new String that contains a subsequence of characters currently contained in this character sequence.
sb.substring(int start, int end) // Returns a new String that contains a subsequence of characters currently contained in this sequence.
sb.toString() //Returns a string representing the data in this sequence.
StringBuffer is thread-safe, sequence of characters. StringBuffer is efficient way of managing String that can be modified.
StringBuilder:
StringBuilder is same set of API of StringBuffer. But StringBuilder to handle modifiable string in single threaded environment. StringBuilder is non-blocking API’s for single threaded environment. It is efficient as well as faster. StringBuilder provides an API compatible with StringBuffer, but with no guarantee of synchronization.
StringBuilder is sequence of characters for non-multithreaded environment. StringBuilder is replacement of StringBuffer for non-multithreaded environment.
In our application is single threaded or we are sure that strings will not be accessed by multi threads, we should go with StringBuilder. It is faster and efficient.
StringBuffer vs StringBuilder:
StringBuilder and StringBuffer has same API and direct replacement based on multithreaded and single threaded environment. Recommended StringBuilder for non-multithreaded environment, StringBuffer for multithreaded environment. What is the different in implementation? The difference is all the methods of StringBuffer implemented with “synchronized” keyword to handle the request from multiple threads.
All the methods of StringBuffer implemented as “synchronized” methods.
public final class StringBuffer implements Serializable, CharSequence, Appendable
{
………………
public synchronized char charAt(int index) // Synchronized to provide consistent access in multi threaded environment.
{
if (index < 0 || index >= count)
throw new StringIndexOutOfBoundsException(index);
return value[index];
}
………………..
}
public final class StringBuilder implements Serializable, CharSequence, Appendable
{
……………………………
public char charAt(int index)
{
if (index < 0 || index >= count)
throw new StringIndexOutOfBoundsException(index);
return value[index];
}
…………………..
}
String vs StringBuffer:
StringBuffer and StringBuilder efficiently manages the string values, it is not creating unnessary string objects. It avoids unnessary immutable string objects by managing with character array.
public final class StringBuilder implements Serializable, CharSequence, Appendable
{
int count;
char[] value; // It is not String, array of characters.
public StringBuilder(String str){
count = str.count;
value = new char[count + DEFAULT_CAPACITY];
str.getChars(0, count, value, 0);
}
}
StringBuffer and StringBuilder manages the string as array of characters. It is not creating immutable Strings in string Constant pool.
Use StringBuffer and StringBuilder for temporary string statement formation,logger statement, updatable string values, large file I/O stream of input processing. Kindly share your valuable thoughts in comments section.