Don’t fill Memory by Strings. Java String and String Constant Pool
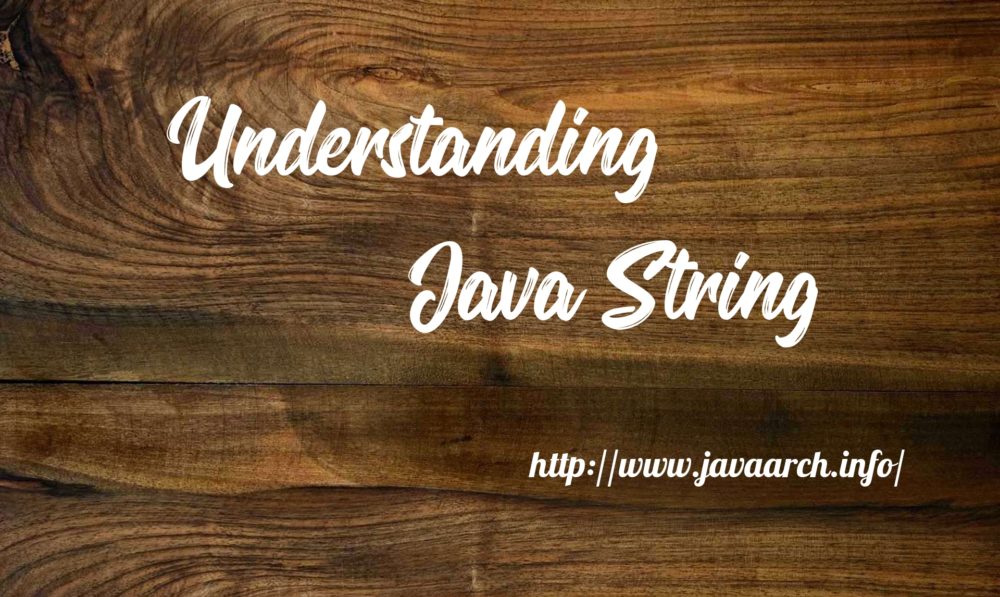
Manipulation of String (Sequence of characters) is most important aspect of programming language. Name, id, address, role and username all manipulated as String in programming world. Java is no different from other language while using the String. In Java, String is sequence of characters and handled to make efficient use of memory. Strings are managed in Java String Pool — the special memory region where Strings are stored by the JVM. In this blog, we would discuss about pros and cons of Java String and understand the String constant pool.
Efficient use of memory leads to overuse of memory without understanding of String implementation in Java
To make Java more memory efficient, JVM sets aside a special area of memory called the “String constant pool.” When the compiler encounters a String literal, it checks the pool to see if an identical String already exists. If a match is found, the reference to the new literal is directed to the existing String, and no new String literal object is created. String literal are managed in String constant pool for reusability. So created string object can’t be changed or modified. Any changes to existing string would create new String. For instance,
String s = “Java” ; // New string “Java” in memory and referred by s
s = s + ” Programming”; // New Strings “Programming” and “Java Programming” in memory. Finally s will refer “Java Programming”
s = s + ” Language”.
System.out.println(s);
It prints “Java Programming Language”. Our intention to print this string. But the above code created 5 String in the pool “Java”, “Programming”, “Language”,”Java Programming”, “Java Programming Language” and finally referred the last formed string in the memory. Remaining all the string are in string constant pool for reusability. It may be reused if same string referred by some other code.
Requires a String, but it created bunch of Strings in memory.
Once string literal created in string constant pool, it would be changed or modified. Any operation on the String object will create a new Object instead of operating on the original content. Let us discuss the memory representation of first 2 lines of the code in the above example,
String s = “Java” ; // New string “Java” in memory and referred by s
s = s + ” Programming”; // New Strings “Programming” and “Java Programming” in memory. Finally s will refer “Java Programming”
Tiny programming mistake would create thousand of String in memory. Write a program to print the multiplication table of number 2.
for(int i = 0; i <=1000 ; i++){
System.out.println(“Multiple of ” + i +” * 2 = “+ i*2);
}
The above tiny program would create at least 2000 String literals in memory. So small programming mistake would fill the memory by string literals. It would cause out of memory exception. Handle the String object with string constant pool in mind. Discussed on internal implementation of String. So when is it appropriate to use String. String object should be used if the value is final and no further update required on that string value like name, id and city.
String is fit for final values and member variables; Not for frequent updatable and temporary statement formation in Java.
Now we are clear on when to use string in java. It is for final values or member variable in a class. Not for temporary statement formation or updatable values.
Here list of string methods and it uses from java documentation. Apache StringUtils provides more robust and handles null in string methods. It provides string reverse and other important string related methods. Will explore Apache StringUtils in upcoming sections.
charAt() Returns the character located at the specified index
concat() Appends one String to the end of another ( “+” also works)
equalsIgnoreCase() Determines the equality of two Strings, ignoring case
length() Returns the number of characters in a String
replace() Replaces occurrences of a character with a new character
substring() Returns a part of a String
toLowerCase() Returns a String with uppercase characters converted
toString() Returns the value of a String
toUpperCase() Returns a String with lowercase characters converted
trim() Removes whitespace from the ends of a String
We are not recommending using string for temporary statement formation, logger statement and updatable values. If it is required string for this use case, what to use to achieve this. There is StringBuilder and StringBuffer to handle that temporary string for logger or display. Upcoming post, we would discuss more on StringBuffer and StringBuilder. Happy Stringing:)