“One and Only for” relationship. Java Inner class & Use of Inner Class
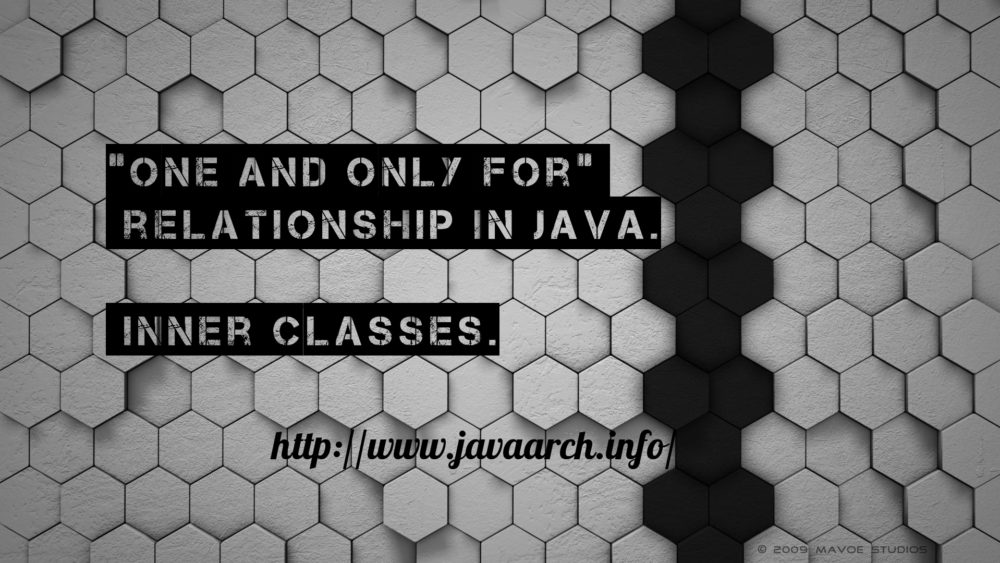
Before start discussion on inner class, let us discuss simple analogy. Boat and Fin(Flipper). Fin exists only for boat an to help boat to move in river. Fin would not be useful if there is no boat. But Fin is not a boat or not an part of boat. But it shares special relationship “one and only for” with boat. To flow smoothly, Boat gives full access to Fin. In short,
Fin is not in “IS-A / HAS-A” relationship with Boat
Boat is not in “IS-A / HAS-A” relationship with Boat
But Fin is “ONE and ONLY FOR” Boat.
Inner classes in java is same as above analogy. Class have relationship of “one and only for” designed as inner classes. Java inner(nested) class is defined inside the body of another class. It is the design proposed for a class is useful only for a single class.
Types of Inner Classes in Java:
Non-static Member inner class – A class created within class and outside method.
Non-static Method inner class – A class created within method.
Static nested class – A static class created within class.
Inner classes not intended to show developer’s Mastery. It is to design the special relationship between classes.
If a class is useful to only one class, it makes sense to keep it nested and together. It is called as Member inner class. For instance, “Calculator” and “Arithmetic” classes. Arithmetic used in Calculator to complete the arithmetic operation. If Calculator class does not require arithmetic class, it is of no use. Arithmetic class only for Calculator. So it is best design to make “Arithmetic” as inner class of “Calculator”.
Calculator has member variables(input) and result(output) variables.
Arithmetic accesses the member variables, does arithmetic calculation and set the output in result of calculator.
Arithmetic is one and only for calculator – Arithmetic can be inner class for calculator.
java nested class example:
package info.javaarch;
public class InnerClasses {
public static void main(String[] args) {
IntCalculator ic = new IntCalculator();
ic.add(10,20);
System.out.println(ic.result);
}
}
class IntCalculator{ //Outer Class
String result;
int number1 = 0 , number2 = 0;
Arithmetic helper = new Arithmetic();
public void add(int no1, int no2) {
number1 = no1; number2 = no2;
helper.add();
}
public void add(int no) {
number2 = no;
helper.add();
}
class Arithmetic{ // Inner class
public void add() {
number1 = number1 + number2;
result = String.valueOf(number1);
}
public void sub() { ………….}
public void mul() { ……….. }
public void div() { ……… }
}
}
- Access modifiers is applicable for class inner classes. We can have private,public and protected access modifier on inner classes.
- Logically without outer class, the inner class is not valid. In the above example, without object of “Calculator”, object of “Arithmetic” is not required. Programmatically, inner class instance can be initiated only if outer class instance exists.
- Inner class can be instantiated outside of outer class. But inner class one and only for outer classes, logically it may not require to instantiate outside of outer class. But it can be done like IntCalculator.Arithmetic confusedArithmetic = ic.new Arithmetic();
- It is same approach in Class Button with ButtonListener,KeyPressListener, KeyDownListener class relationships. All the listeners one and only for “Button” class. So it makes sense to design all the listener as inner classes.
- Inner classes provide high level of abstraction. The inner class implementation is hidden and only outer class methods are exposed.
- Inner classes provides high level of encapsulation. Instead of writing all the key down methods, button listener methods in single class “Button”, it designed to have multiple classes ButtonListener,KeypressListener and KeyDownListener. All listener handles it respective events and methods. All the listeners and outer classes behaves only what is expected from that object. No unrelated method in any of the classes.
Method Inner Class:
In Java, we can write a class within a method. The method scope of the inner class is restricted within the method. It is used to group of the block of code with class name for encapsulation. For instance, sub() method to subtract two numbers. It should not be allowed to do other than subtract operation. But we want to print the result, we may require to add the print statement. It is against design to add print statement respect to encapsulation design aspect. But to follow the standard create method inner class and do the print action. It ensures encapsulation and it highly affect code reusability. Use if your code really require to have method inner class.
public void sub() {
number1 = number1 – number2;
result = String.valueOf(number1);
class DisplayResult{ //Inner class
public void display() {
System.out.println(“Result is ” + result);
}
}
A method-local inner class can be instantiated only within the method where the inner class is defined.
We discussed simple class member inner classes(Non-static inner classes). Now I hope you are sure about what is inner classes,nested classes and usage of inner classes. Next section we would discuss on actual usage of static inner classes. Happy Learning.:)