Java Best Coding Practice is 80 Characters per Line. Use Static import.
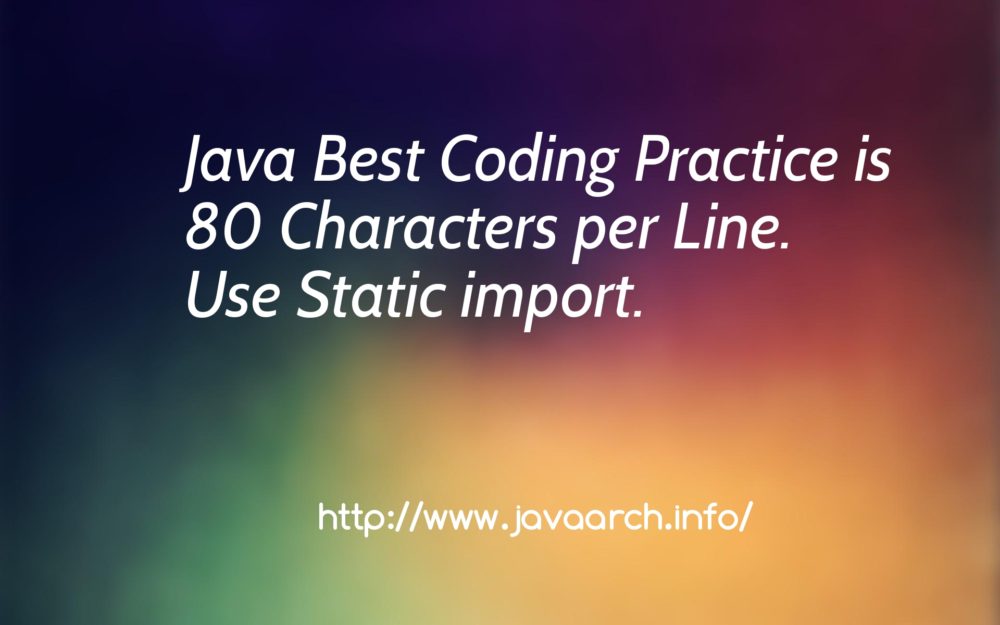
Java coding practices recommended that maximum 80 characters in single line of the code. It is best for readability and understand the code. If our class require static members in more than 20 lines of our code and all lines are more than 80 characters in length, static import would help us to reduce the length of the line. Static import allows to access the static member of a class directly without using the class name(ie fully qualified name). In simple terms, we are importing the class static members instead of class. So class static members can be accessed without class name using static import.
Let us take an example of libraryconstants class.
package info.javaarch;
public class LibraryConstants{
public static final int NUMBEROFBOOKSALLOWED = 2;
public static final int LATEFINE = 10;
public static final int MAJORDAMAGEPENALTY = 100;
public static final int MINORDAMAGEPENALTY = 20;
public static final int YEARLYSUBSCRIPTIONFEES = 1000;
}
package info.javaarch;
import static info.javaarch.LibraryConstants.*; //Static import of static member of class LibraryConstants
public class StaticImportExample {
public static void main(String[] args) {
System.out.println(“Total Fine: “+ calculateFine(2,true,10));
}
public static int calculateFine(int books,boolean isMajorDemage,int days) {
int fine = days * books * LibraryConstants.LATEFINE + books * LibraryConstants.MAJORDAMAGEPENALTY; //107 characters
int fine1 = days * books * LATEFINE + books * MAJORDAMAGEPENALTY; // same line as above with 73 characters using static import.
return fine;
}
}
We reduced the character count from 107 to 73 using static import. Avoid code line longer than 80 characters.
AVOID CODE LINE LONGER THAN 80 CHARACTERS
If an expression will not fit on a single line or worst case that more than 80 character in single line, it should be broken according to these general principles:
- Break after an logical group such as ().
- Break after an operator.
- Start an next line with 4 space indent like below,
int fine = ( days * books * LibraryConstants.LATEFINE ) +
( books * LibraryConstants.MAJORDAMAGEPENALTY );
- Having code with 80 lines of characters fit in device visible screen area and easy to read. It would improve the readability of the code and help to understand the code better by missing the code logic in out of visible screen area.
- Use static imports only if static members required in more than 10 lines and lines length go beyond 80 characters.
- Logically static imports should be used only to import the static members created by you. Like LibraryConstants example created by us and imported into StaticImportExample class.
- Avoid using static import for java standard libraries and third party libraries. It makes the code complex to read and less readable. We can’t differentiate the static import member and our own class members by name. For example,
package info.javaarch;
import static java.lang.Math.max;
public class StaticImportExample {
public static void main(String[] args) {
System.out.println(max(10,20)); // Java Math library method max.
System.out.println(maximum(10,20)); // Our method maximum. Both been called in same way. Not able to differentiate
}
public static int maximum(int a, int b) {
return a > b ? a : b;
}
}
You can apply static import statement on static members (static fields and static methods). Do not create interfaces only to have constants(constant interfaces). Interfaces are powerful and using interfaces for constants would introduce Constant Interface Antipattern. Constant Interface Antipattern is creating interface only to have constants. Avoid by create common constants in class and import statically to your classes.
Logically static import imports the static member to the class. Access the static members like the class static members. So it should be used only to import static member created by us. Do not use static import for java standard libraires, it may not require as all the java standard libraies class name are short like Math, System and Long. Use static import if it satisfies all teh above coding standards,otherwise use fully qualified name of the static members. Happy import:)