Mark the Method Parameters Read-Only or Read-Write using “final”
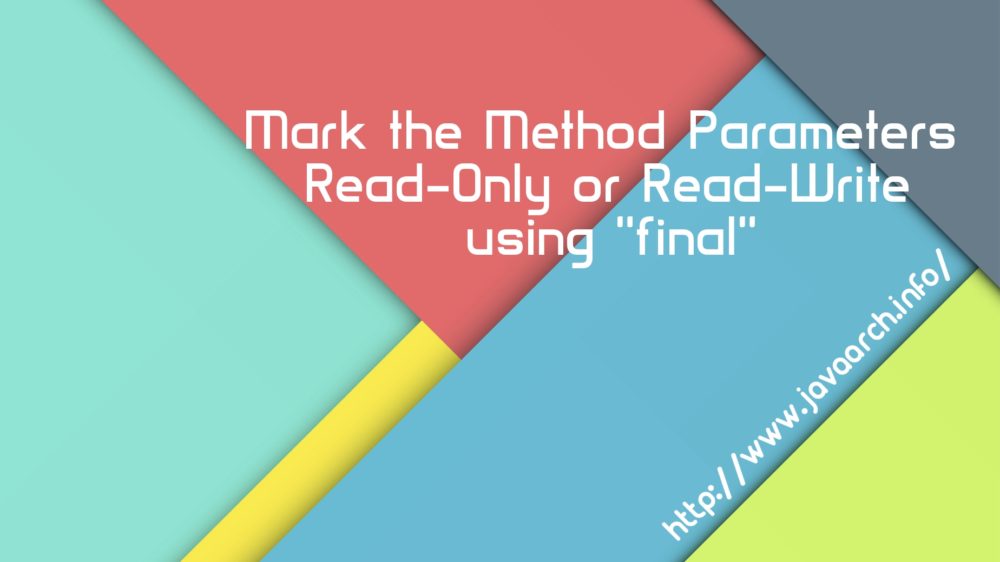
Java methods accept parameters, execute business logic and return results. We can pass multiple argument to the methods and it return only one value from the methods. Some real time scenario, we may require to get more than one return values from single method. To get two return value from an method, one return value goes as input argument and another one return as a result.(Actually programming practices not encourage this design. We are considering this scenario to handle exceptional requirement.) Now we decided to pass two set of parameters to a method. One set of parameters are actual input for processing, another set of parameters are to get as a result from method. This design implemented and it behaved as we expected. Now the real confusion starts. How can we identify the actual set of parameters and result return parameters logically. In another words, Read-Only parameters vs Read-Write parameters of the methods. Let us discuss on “final” parameter of method on this topic.
The final keyword on a parameters restricts the parameters can not be reassigned inside the method. In case of primitive parameters, the value can’t be changed. In case of reference parameters, the object can’t be reassigned with new argument, but the values inside reference object can be modified. Reference can’t be changed, but reference members can be changed even we mark the parameter as “final”. This all related to how final behaves in method parameters.
But logically if method parameter is “final”, method developer conveys that parameter is “Read-Only”. It means that is not changed it reference and reference members both. It gives clarity and method impact on parameters to other team members. It would increase utilization of the method as method parameters definition clarified it impact. Mark the parameter as “final” communicates developer’s design and intention to team members.
“final” keyword conveys developer’s message to team members.
package info.javaarch;
import java.util.ArrayList;
import java.util.List;
public class FinalExample {
public static void main(String[] args) {
Employee emp1 = new Employee(“James”,33);
Employee emp2 = new Employee(“David”,21);
Employee emp3 = new Employee(“Charles”,43);
Employee emp4 = new Employee(“Mark”,87);
Employee emp5 = new Employee(“Steven”,67);
Employee emp6 = new Employee(“Kevin”,65);
ArrayList<Employee> empList = new ArrayList<Employee>();
ArrayList<Employee> seniorEmpList = new ArrayList<Employee>();
empList.add(emp1); empList.add(emp2); empList.add(emp3);
empList.add(emp4); empList.add(emp5); empList.add(emp6);
int count = Utility.getSeniorEmpCount(empList, seniorEmpList); // returns two result. One is count and another one is seniorEmpList.
System.out.println(“Count:” + count);
}
}
class Employee{
String name;
int age;
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
}
class Utility{
// This method returns 2 values,one is count and one is list of senior employees in input parameter seniorEmpList
public static int getSeniorEmpCount(final List<Employee> empList, List<Employee> seniorEmpList) {
int count =0;
for(Employee e : empList) {
if(e.getAge() > 60) {
seniorEmpList.add(e);
count++;
}
}
return count;
}
}
Marking method as “final” is a decision of implementation developer. No one can force or restrict the usage of “final” in method parameter except implementor. Even Java and inheritance does not force them to do that. If you declare method parameters final in your interface or in parent class, people implementing that interface don’t need to declare them final, and can modify them in their implementations. The final keyword is not part of the method’s signature, it can be removed from its overriding method in its subclass.
Programming is a skill best acquired by practice and example rather than from books.
Example of final class and member variables of previous post,
package info.javaarch;
public class FinalClassExample {
public static void main(String[] args) {
InterestCalculator ic = new InterestCalculator();
float si = ic.calculateSI(1000f,8.7f,10f);
System.out.print(“\nSimple Interest = ” + si);
}
}
final class InterestCalculator{
private final Integer HUNDRED = 100;
public float calculateSI(float principal,float rate, float years) {
return principal * rate * years / HUNDRED;
}
}
We discussed “final” keyword intention and implementation. I hope all the doubts related to final discussed in details. Do not say “final” is one of the keyword in java, it is useful for developer design. Start using “final”. Happy Learning.!!!