Writing comments in java and Generating document by “javadoc”.
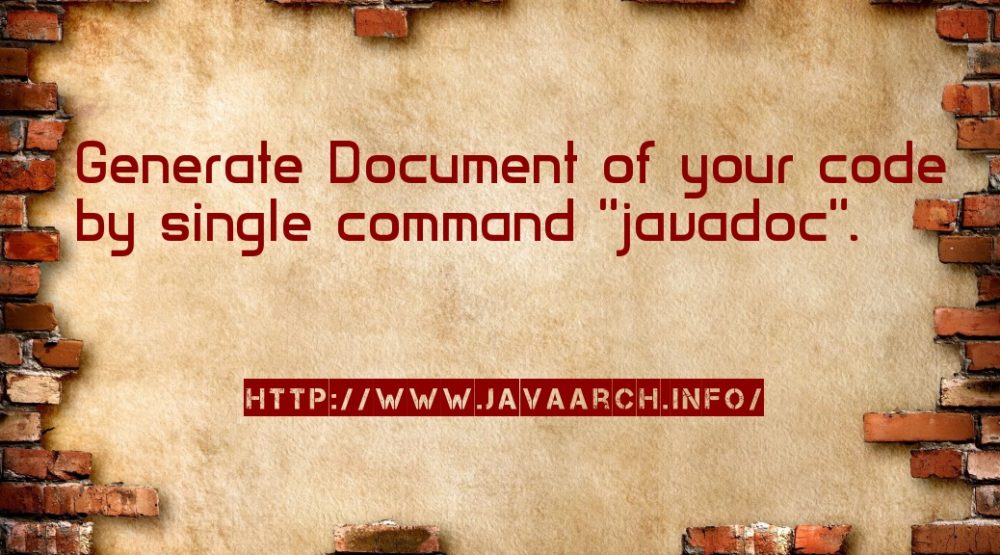
The Java language supports three types of comments in code to explain the business logic in code, to explain the algorithm logic to other developers in code itself or to generate the document content in coding itself.
- Single line comments : //………………….
- Multi line comments : /* ………………. */
- Documentation comments /** Document Content */
Comments to Developers:
Software applications or solutions are developed in 1 years and maintained for 9 years.
Typically maintenance consumes 40 to 80% of software costs and effort.
Application developed by 10 developers maintained by 100 developers.
From above industry observations, we can understand scope of software maintenance. So documenting the complex business logic and purpose of the method should be documented properly. Otherwise code can not be understandable by other developers. and it makes developers life worst. We could see sometimes we could not understand our code logic if we look at our code after some years. So better to document business logic and assumption in code.
Single line comment:
Use single line comment to write one line about the method or explain a business logic in single line. The format of the single line comment is (//). Line of code starts with “//” are considered single line comments.
//It displays the object values in readable form. => Single line comments in code.
public String toString(){
…………
}
Multi line comments:
Use multiline comments to write about business logic, algorithm used or complex logic in the code. We can write more than one line of comments between /* …… */.
int number1 = 10;
int number2 = 20;
/* number1 assigned addition of two swap integers,
* number2 and number1 swapped by arithmetic operation.
*/
number1 = number1 + number2;
number2 = number1 – number2;
number1 = number1 – number2;
System.out.println(number1 + ” : ” + number2);
Comments are important in code for better readability and easy to use. But do not write comment for each line of the code. Write comments only for the code which has business logic or complex handling.
Documentation for API users :
Documenting all the methods of the projects is required to consume the methods. Java provides javadoc utility to generate formatted the HTML document for API users. Javadoc should be written in this format(/**…..*/) in the code for a project/software package. Javadoc uses these comments to generate a documentation page for reference, which can be used for getting information about methods present, its parameters,return type, description and throwable exception.
Code and respective document content at single place.
/* …… */ => This is multiple line comments.
/** ……. */ => This is javadoc comments.
/**
* It divides two integers and returns the results.
* @param a Integer
* @param b Integer
* @return
*/
public Integer div(Integer a,Integer b) {
return a % b;
}
Generating the java doc for projects:
The JDK javadoc tool uses doc comments when preparing automatically generated documentation. It gives flexibility of having code and respective code documentation at one place. It is easy to maintain the code and document. It gives flexibility to update the document if any change in the code.”javadoc” is bundled in JDK. After intall the jdk, javadoc utility can be found in bin folder.
Below command generates the HTML document of “Calculator” class in CalculatorDoc folder. Generate Document of your code by single command “javadoc”.
C:\Program Files\Java\jdk1.8.0_161\bin>javadoc.exe Calculator.java -d /CalculatorDoc
Loading source file Calculator.java…
Constructing Javadoc information…
Creating destination directory: “/CalculatorDoc\”
Standard Doclet version 1.8.0_161
Building tree for all the packages and classes…
Generating \CalculatorDoc\Calculator.html…
Generating \CalculatorDoc\package-frame.html…
Generating \CalculatorDoc\package-summary.html…
Generating \CalculatorDoc\package-tree.html…
Generating \CalculatorDoc\constant-values.html…
Building index for all the packages and classes…
Generating \CalculatorDoc\overview-tree.html…
Generating \CalculatorDoc\index-all.html…
Generating \CalculatorDoc\deprecated-list.html…
Building index for all classes…
Generating \CalculatorDoc\allclasses-frame.html…
Generating \CalculatorDoc\allclasses-noframe.html…
Generating \CalculatorDoc\index.html…
Generating \CalculatorDoc\help-doc.html…
Here the Calculator.java and generated document of Calculator.java for your reference.
CalculatorDoc Calculator
Look at the document to know how well formatted and easy to use documentation for our class by javadoc utility. Even if you are not sure about consumer or API users, write documentation comments for all the methods with description and parameters. Highlight of this document comment is you can document your name as @author annotation. Your name will follow wherever or whoever access the code.
Points to remembers:
- Use single line comment to write one line comment in middle of the code .
- Use multi line comments to explain complex logic or algorithm logic in the code.
- Use document comments to generate detailed document for your code.
Java comments are powerful way to convey to logic to other in the code. It make your junior programmer or maintenance engineer life easy. Documentation comments give flexibility to write code and document in single place. Kindly do not use commenting operator(// or /* */) only to comment out your unused code or unwanted code . It is powerful if you use it proper way. Happy documentation !!:)