Writing a class and creating object in Java
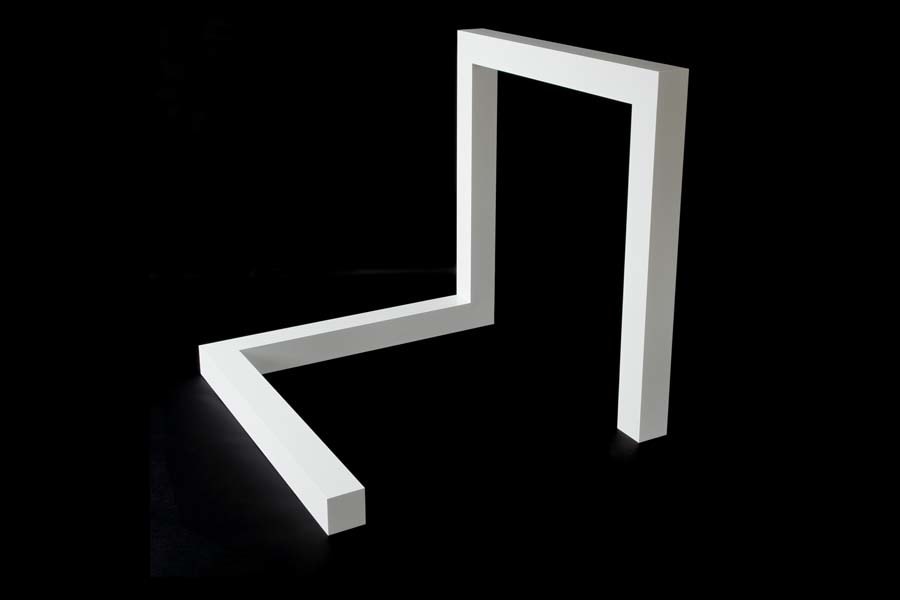
A class is a blueprint or a template for creating objects and defines its properties and behaviors. Java class objects exhibit the properties and behaviors defined by its class. A class can contain fields and methods to describe the behavior of an object.A class is a blueprint or a template for creating objects and defines its properties and behaviors. Java class objects exhibit the properties and behaviors defined by its class. A class can contain fields and methods to describe the behavior of an object.
public class Cube {
private int length;
private int breadth;
private int height;
public int getVolume() {
return (length * breadth * height);
}
//all getter and setters of instance variables
}
The above cube class would look fine and return volume of the cube. Writing a class would be as simple as that. But writing class in a sensible and useful design would be significant in architecture design. Just think of constructor of the class,
public class Cube {
private int length, breadth, height;
public Cube(int l, int b){
length = l ; breadth = b ;
}
public Cube(int l, int b,int h){
length = l ; breadth = b ; height = c;
}
………………..
}
The above class definition with constructor would compile without any issue. But just think of object creation as,
Cube cube1 = new Cube (10, 20);
Cube cube2 = new Cube (10, 20, 30);
Cube1 with length and breadth without height. Is it cube? cube without height never been called as cube. So do not give or expose constructor which allows object construction in invalid or illogical objects.
“Always construct object in valid state by restricting the constructor.”
Java emphasize on object oriented programming (OOPS). Data hiding and data encapsulation should be done in code to achieve OOPS. Mark all your instance variable as private and expose them by accessor methods.
“All the instance variable should be private and expose by accessor methods.”
This minimizes coupling between objects and increase the cohesiveness in the design. Doing so ensures the consistency and enhances program maintainability.Single responsibility principle is dictated for class design. The principle expects “a class should have responsibility over a single part of the functionality provided by the software; should have one, and only one, reason to be changed.” If we design the class with this principle in mind, a class should not go beyond more than 900 lines of code.
“Smaller must be better: A class should contain an average of less than 30 methods, resulting in up to 900 lines of code.”
While writing class, we have to log the object values in readable form. Logging the object writes some values and it is not what we expect it. We expect logging the object with proper instance variable. How to do that? Do you know that all the java class extends java.lang.Object by default. The java.lang.Object class is the ultimate superclass of all objects If a class does not explicitly extend a class, then the compiler assumes it extends java.lang.Object.
public Object()
public final Class getClass()
public int hashCode()
public boolean equals(Object obj)
protected Object clone() throws CloneNotSupportedException
public String toString()
public final void notify()
public final void notifyAll()
public final void wait(long timeout) throws InterruptedException
public final void wait(long timeout, int nanoseconds) throws InterruptedException
public final void wait() throws InterruptedException
protected void finalize() throws Throwable
public class Cube {
private int length, breadth, height;
public Cube(int l, int b){
length = l ; breadth = b ;
}
public Cube(int l, int b,int h){
length = l ; breadth = b ; height = c;
}
//getters and setters, constructor
@Override
public boolean equals(Object o) {
if (o == this) return true;
if (!(o instanceof Cube)) {
return false;
}
Cube cube = (Cube) o;
return this.length == o.length && this.breadth == o.breadth && this.height == o.height ;
}
//Idea from effective Java : Item 9
@Override
public int hashCode() {
int result = 17;
result = 31 * result + o.length;
result = 31 * result + o.breadth;
result = 31 * result + o.height; return result;
}
@Override
public String toString() {
return “Length: + o.length +”,Breadth:”+o.breadth+”,Height:”+o.height);
}
}
Override toString() to log or print the object values in readable or meaningful form.Override equals() to compare the object by its properties and not by it memory location. Remember comparing to Cube object with same height, breadth and length should return true always. overriding equals() method is required for logical comparison.
Override hashCode() as it required to achieve the real power of HashSet and HashTable feature in java. Stay tuned. Would discuss more on hashcode in Collection. Here consider the blind rule that override hashCode() for all the classes.
“Always override equals, hashCode and toString methods of Object class”