java static variables and static methods
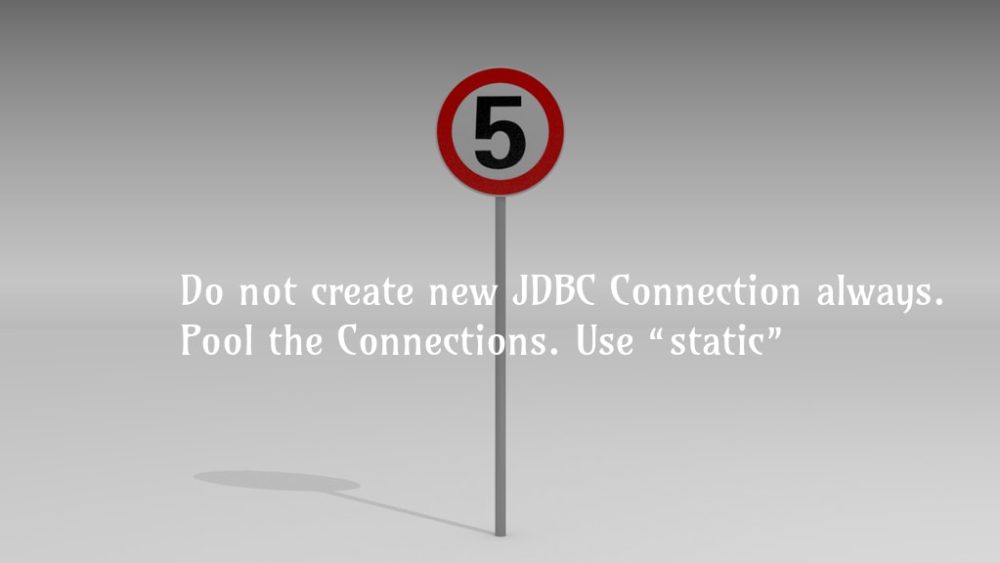
Static members help to write code to support sharing of objects or to create only one instance of class (Singleton pattern) in application. Let us discuss what static member in Java. Static members are class variables and methods with modifier “static”. Static members are called class variables and class methods.
Static members are class level variables and class level methods.
All the static members initialized when creating class in JVM. Just note the point, all the static variables and static methods initialized during creation of class. Do you think any instance exist for that class at that time? Surely no. We would not have any instance of the class while initialization of the static blocks. Do you think accessing instance (non-static) variable or method would be valid inside static block? No. Accessing the instance variable or instance method inside static block or method is invalid and not allowed.
Accessing non-static members in static methods is invalid.
Now just reverse the scenario. All the static variables and methods are initiated for the class. Now we try to create instance of the class. Now instance, static variable and static methods are initialized and all in valid state. Do you think accessing static members from non-static (instance) members is invalid use case? No. It is valid use case. We can access the static members from non-static methods.
Static members can be accessed from non-static methods.
Sharing of object:
Usually we write the logger message in a log file to mark the workflow in our application. We would create logger to write the log message into a file. If we have 1000 of classes, creating logger object in all the classes and each logger object try to write to same file. The log file would not be in readable. Each logger object would try to open the same log file and establish file I/O stream. We have to write code to handle the streams in all the classes. How complicate this simple log collection? Now just think of single logger object would write the logs to single file. All the classes should use the single logger object to write into file. No more logger object allowed. Only one logger object to write it.
class Logger {
public static String logfile=”serverlog.log”;
public static void log(String message) {
//… write into log file “serverlog.log”
System.out.println(“Message:”+ message);
}
}
class TaxCalculator {
void display() {
Logger.log(“Tax calculated successfully.”); // Logger.log
}
}
class TaxDeductor {
void display() {
Logger.log(“Tax deducted from account.”); // same Logger.log
}
}
Singleton Pattern:
In application architecture, we may have to restriction only one object for few classes. Creating multiple object of classes would be costlier such as payment gateway, external connectors. Singleton pattern restricts the instantiation of a class and ensures that only one instance of the class exists in the java virtual machine. “static” members gives us provision to implement singleton pattern in java.
public class PaymentGatewayConnector {
private static PaymentGatewayConnector connector = null;
public static PaymentGatewayConnector getInstance() {
if(connector == null) {
connector = new PaymentGatewayConnector ();
}
return connector;
}
}
Connection pooling with static:
public class ConnectionPool {
private static JDBCConnection[] jdbcConnections = null;
private static Boolean[] isConnectionBusy = null;
public static void createConnections() {
if(jdbcConnections == null) {
jdbcConnections = new JDBCConnection[5];
isConnectionBusy = new Boolean[5];
}
}
public static JDBCConnection getConnection() {
for(int i=0;i<jdbcConnections.length;i++){
if(!isConnectionBusy[i]){ return jdbcConnections[i];
return “null”;
}
}
}
The static modifier is used to create variables and methods that will exist independently of any instances created for the class. All static members exist before you ever make a new instance of a class, and there will be only one copy of
a static member regardless of the number of instances of that class. In other words, all instances of a given class share the same value for any given static variable. We’ll cover static members in great detail in the next chapter.
Things you can mark as static:
■ Methods
■ Variables
■ A class nested within another class, but not within a
■ Initialization blocks
Things you can’t mark as static:
■ Constructors (makes no sense; a constructor is used only to create instances)
■ Classes (unless they are nested)
■ Interfaces
■ Method local inner classes
■ Inner class methods and instance variables
■ Local variables
Key Takeaways:
“static” is not just another modifier in Java world. It is for sharing objects between instances, help to implement connection pooling and singleton pattern. Use it wherever it required.