Java naming conventions
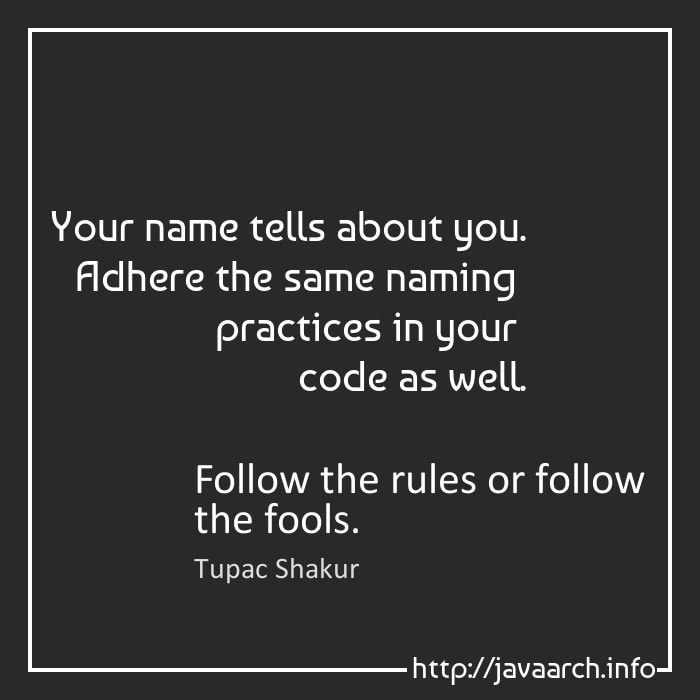
“A name represents identity, a deep feeling, and holds tremendous significance to its owner.”
Naming the code artifacts is important in programming coding practices. In Java, we would name the variables, methods, constants, classes and interfaces. We have to follow the standard naming conventions for each pieces. Proper and meaningful names helps others to understand the application and use of the objects. It enhances the clarity and simplicity of the program.
A variable provides us with named storage that our programs can manipulate. Every variable is assigned a data type which designates the type and quantity of a value it can hold. Constants are values that will not be modified once they have been assigned to a variable.
A method is a set of code which is referred to by name and can be called at any point in a program simply by utilizing the method’s name. Each method has its own name. When that name is encountered in a program, the execution of the program branches to the body of that method. When the method is finished, execution returns to the area of the program code from which it was called, and the program continues on to the next line of code.
On top of this, we would have the main artifacts such as packages, interfaces and classes in our application. Every artifacts has specific purpose and functionality attach to it. The intention of the classes,methods and interfaces should be identified by its names. It is developer responsibility to name the variables, methods name properly.
“Use Meaningful & Familiar names for classes, methods, interfaces and packages”.
“Avoid long names and don’t use same names that differs only in lowercase and uppercase characters”
The above 2 points are common for all the programming language and not only for Java. Related to Java, Oracle recommends for naming the methods and classes.
Naming for Classes and Interfaces:
The first letter should be capitalized and if several words are linked together to form the name, the first letter of the inner words should be uppercase.(camelCasing). For example, class name should be “Person”, “Employee” or “ReportGenerator”. For interfaces, the name should typically by adjectives like Runnable, ActiveListener.
Naming for Methods and Variables:
For method names, first letter should be lowercase, and then normal camelCase rules should be used. The method name should represent action and verb-noun pair. For variable name,the camelCase format should be followed, starting with a lowercase letter.
Constants can be created by marking the variable as static final. The name of the Constant should be uppercase letters with underscore character as separators.
Naming for Packages and JavaBeans accessor methods:
The package names should be reversed, lowercase form of your organization’s internet domain name as the root qualifier for your package name. For example,
“package info.javaarch.beans”.
JavaBeans is a class which is created for sharing to other tier in the software architecture. For instance, the service layer would get the information from third party vendor and send the javabean object to component layer in the n-tier architecture. IT provides the easy maintenance and simplicity in sharing the object between different tiers in the enterprise architecture. Simple rule for JavaBeans is, it is simple class with no-arg constructor and implements Serializable interface.
JavaBeans specification establishes standard names conventions for methods that give access to the properties of the class and interfaces. A JavaBean exposes boolean properties using methods that begin with “is”. A JavaBean gives read access to other property types using methods that begin with “get”. A JavaBean gives write access to the properties using methods that begin with “set”.
Would have more discussion on naming convention and javabeans convention with one example,
package info.javaarch.bean; //Reversed the domain name “info.javaarch”
public class Student implements Serializable { //JavaBean “Student” ; implements Serializable and accessor methods.
private String name;
private int rollNo; // Variable name starts with lowerCase
Student(String name, int rollNo){
this.name = name;
this.rollNo = rollNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getRollNo() {
return rollNo;
}
public void setRollNo(int rollNo) {
this.rollNo = rollNo;
}
}
public class Course implements Serializable {
private int id;
Student(int id){
this.id = id;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
// ” info.javaarch.service” and info.javaarch.component” are two different packages.
// The communication between both the layer by JavaBeans objects.
package info.javaarch.service;
import java.util.List;
public interface StudentDBConnectable { //Interface name as adjective
public static final MAX_CONNECTION_TO_DB = 10; //Constant name with uppercase and seperated by “_”.
public List<Student> getAllStudents(Course c);
}
package info.javaarch.service;
import java.util.ArrayList;
import java.util.List;
public class StudentDaoImpl implements StudentDBConnectable {
//list is working as a database
List<Student> students;
//retrive list of students from the database
@Override
// Gets JavaBean(Course) object as argument and Returns Javabean(Student) as Result.
// The service and component layer integration and data transfer only by JavaBeans. Follow the same design architecture in all the layer of enterprise application.
public List<Student> getAllStudents(Course c) {
//Connect to the database and get all the student enrolled for the course
//Iterate all the student create list
return students;
}
}
package info.javaarch.component;
public class DaoPatternDemo {
public static void main(String[] args) {
StudentDBConnectable studentDao = new StudentDaoImpl();
Course c = new Course();
c.setId(100);
//print all students
for (Student student : studentDao.getAllStudents(c)) {
System.out.println(“Student: [RollNo : ” + student.getRollNo() + “, Name : ” + student.getName() + ” ]”);
}
}
}
We have discussed all the naming convention and the use of javabeans in the enterprise architecture. I hope it gives clear explanation on both the section. Kindly share more thoughts on this topics. Happy Reading…!! Enjoy Learning…!!!