Constructors in Java
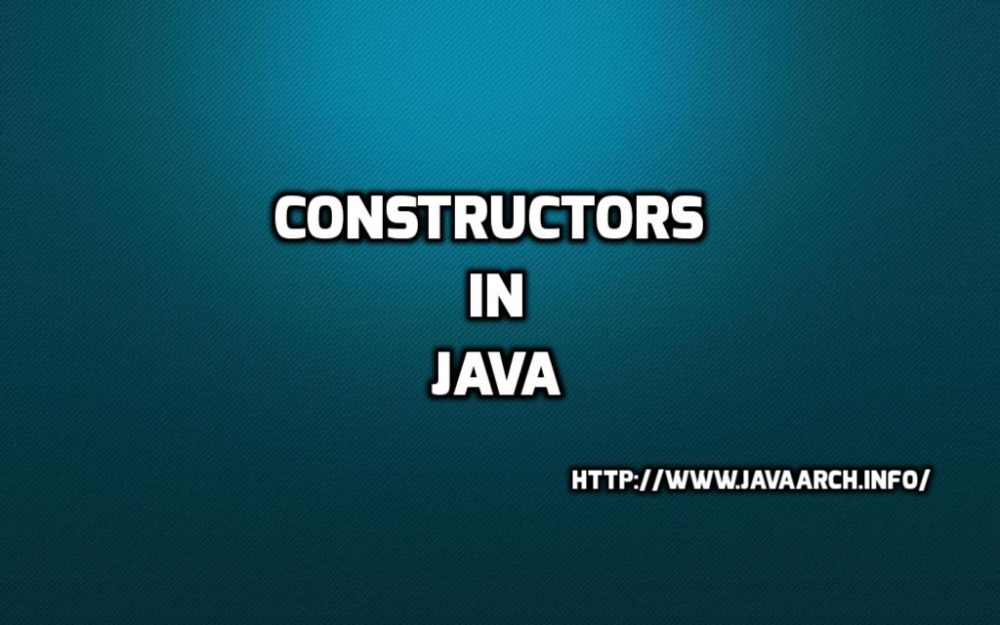
A constructor in Java is a block of code similar to a method that’s called when an instance of an object is created. Constructors are used to initialize the object’s state. Like methods, a constructor also contains collection of statements that are executed at time of Object creation.A constructor in Java is a block of code similar to a method that’s called when an instance of an object is created. Constructors are used to initialize the object’s state. Like methods, a constructor also contains collection of statements that are executed at time of Object creation.
A constructor doesn’t have a return type. The name of the constructor must be the same as the name of the class. Unlike methods, constructors are not considered members of a class. Each time an object is created using new() keyword at least one constructor (it could be default constructor) is invoked to assign initial values to the data members of the same class. Rules for writing Constructor: A constructor in Java can not be abstract, final, static and Synchronized. Access modifiers can be used in constructor declaration to control its access i.e which other class can call the constructor.
Let us discuss practical aspect to the constructors. Atleast one constructor would be called by default. So we can use constructor to restrict the creation of invalid objects. Let us discuss with example of object of Employee.
public class Employee{
String empID;
String empName;
…………………..
}
Let us create object for employee as Employee emp1 = new Employee(); // It would compile and creates invalid objects. It invokes the default constructor and It creates employee without emp ID, emp name. Do you think any employee without employee properties. It is invalid object and constructor provides way to restrict the invalid object creation. Just enhance the “Employee” with constructor with 2 argument constructor.
public class Employee{
String empID;
String empName;
public Employee(String id, String name){
empID = id; empName = name;
}
}
Employee emp1 = new Employee(); // It would throw compilation error
All the required variable should be assigned in constructor ;Restrict invalid object creation by constructor Constructor overloading
Constructor can be overloaded as it differ by number of argument or argument type. In “Employee” object, if employee has passport information, we have to send passport detail in constructor.
public class Employee{
String empID;
String empName;
String passportNo;
public Employee(String id, String name){
empID = id; empName = name;
}
public Employee(String id, String name, String passportInfo){
//Constructor overloading this(id,name); passportNo = passportInfo;
}
}
Constructor overloading would be helpful to create object with different set of initial arguments.
Too many constructor is not good; Create a Builder object
If class has more number of attributes, the code becomes increasingly harder for clients if it has multiple constructor. Which constructor should I invoke as a client? The one with 2 parameters? The one with 3? What is the default value for those parameters where I don’t pass an explicit value? What if I want to set a value for address but not for age and passportNo? In that case I would have to call the constructor that takes all the parameters and pass default values for those that I don’t care about. Additionally, several parameters with the same type can be confusing. Was the first String the passportNo or the address? Let us discuss on builder pattern. A builder class should have all the attributes of the class. However, given the fact that the builder class is usually a static member class of the class it builds, they can evolve together fairly easy.
A single builder can be used to create multiple objects by varying the builder attributes between calls to the “build” method. The builder could even auto-complete some generated field between each invocation, such as an id or serial number. An important point is that, like a constructor, a builder can impose invariants on its parameters. The build method can check these invariants and throw an IllegalStateException if they are not valid. It is critical that they be checked after copying the parameters from the builder to the object, and that they be checked on the object fields rather than the builder fields.
package com.test;
public class TestProgram {
public static void main(String[] args) {
Employee.EmployeeBuilder builder = new Employee.EmployeeBuilder(“EMPID1”, “John”).address(“USA”).passportNo(“980900000”); // Constructor with 4 parameters Employee user1 = new Employee(builder);
builder = new Employee.EmployeeBuilder(“EMPID2”, “Jack”).passportNo(“9809876000”); // Constructor with 3 values filled
Employee user = new Employee(builder);
}
}
class Employee {
private final String empID;
private final String name;
private final int age;
private final String passportNo;
private final String address;
Employee(EmployeeBuilder builder) {
this.empID = builder.empID;
this.name = builder.name;
this.age = builder.age;
this.passportNo = builder.passportNo;
this.address = builder.address;
}
// Getter and setter methods for instance variables
public static class EmployeeBuilder { private String empID; private String name; private int age; private String passportNo; private String address;
public EmployeeBuilder(String empid, String name) { this.empID = empid; this.name = name; }
public EmployeeBuilder age(int age) { this.age = age; return this; }
public EmployeeBuilder passportNo(String passportNo) { this.passportNo = passportNo; return this; }
public EmployeeBuilder address(String address) { this.address = address; return this; }
public Employee build() { return new Employee(this); }
}
}
Do not allow to create instances for Utility Class:
Utility classes provide static methods for calculations. It will process the inputs and return results. To access the static method, class is sufficient and instance in not required. Creating instances can be blocked by throwing the exception in constructor.
class Utility{
public class Utility() {
throw new Exception(“Instance is not requried.”);
}
public Int findMax(int a, int b){……………..}
public int findMin(int a, int b){………..}
}
Points to remember:
- All the required variable should be assigned in constructor ;Restrict invalid object creation by constructor
- Overload the constructor(Max 3 constructor)
- Too many constructor is not good; Create a Builder object
- Do not allow to create instances for Utility Class
Consider all these factor before write an constructor for the class. We have more to discussion on number of instance for the class with design patterns such as singleton pattern, Factory pattern. Will discuss more on next topic. Happy Reading.