Abstract Class in Java
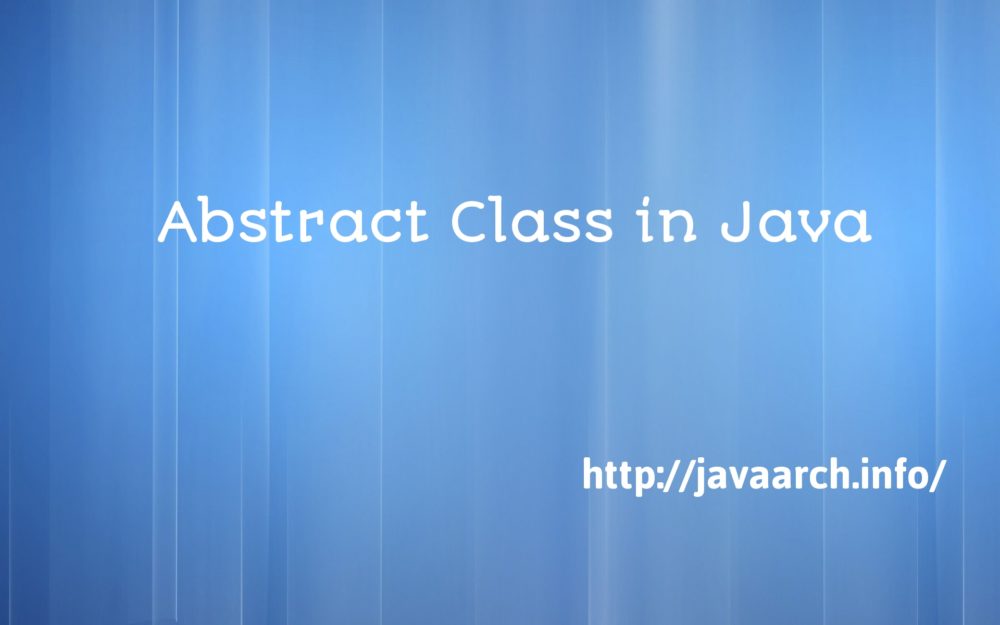
An abstract class’s purpose is to provide an appropriate super class from which other classes can inherit and thus share a common design. Abstract class would be created to share code among several closely related classes. All the sub-classes extend the abstract class would get all the common methods or fields available in the super class. Abstract class allows concrete methods as well, it does not provide 100% abstraction and it provides partial abstraction.
Abstract classes are incomplete and partial implementation of the common methods. Instance of Abstract class does not have any real meaning and it is not allowed. Abstract class can’t be instantiated. An abstract class can not be instantiated and it is not allowed to create an object of it. Let us discuss with an example,
package info.javaarch;
import java.util.List;
// Abstract Super class
abstract class Insurance {
String planId;
String planName;
String companyName;
int premiumAmount = 0;
double premiumTax = 0;
//……
public void printInsuranceInfo() {
System.out.println(” Plan name:” + planName + “,plan id:” + planId);
}
public abstract void addPremium(int amount); // Abstract Method
}
//Sub class 1
class MedicalInsurance extends Insurance{
List<String> diseaseList; //List of diseases covered in this policy
//…………
@Override
public void printInsuranceInfo() {
System.out.println(” Plan name:” + planName + “, plan id:” + planId + “, number of diseases covered” + diseaseList.size());
}
@Override
public void addPremium(int amount) { // Implementation of Abstract method
premiumTax = premiumTax + (amount* 0.01); // One percent of amount would be increased in premiumTax
}
}
//Sub class 2
class VehicleInsurance extends Insurance{
List<String> vehiclePartsList;
//…….
@Override
public void printInsuranceInfo() {
System.out.println(” Plan name:” + planName + “, plan id:” + planId + “, vehicle parts covered” + vehiclePartsList.size());
}
@Override
public void addPremium(int amount) { // Implementation of Abstract method
premiumTax = premiumTax + (amount* 0.01); // One percent of amount would be increased in premiumTax
}
}
In the above example, Insurance is abstract superclass. It is not allowed to create object for abstract class as it does not have complete design. The first concrete class in the inheritance tree gives complete implementation of all the abstract methods and provides complete class.
Common implementation of Abstract classes and methods:
An abstract class has no use until unless it is extended by some other class.
Abstract method exists in a class, the class must be abstract as well.
Abstract class can have constructors as same as other classes.
It can have non-abstract method (concrete) as well.
Abstract method has no body and always end the declaration with a semicolon(;).
Abstract method must be overridden in the first concrete class in the inheritance tree. An abstract class must be extended and in a same way abstract method must be overridden.
Use abstraction to improve code re-usability and share the methods/variables between multiple related classes. In Code refactoring technique, Pull up method technique encourages to have all the common method in the super class to avoid duplication of code in multiple classes.
Multi Level Inheritance In Java:
Multilevel inheritance is a design of subclass is inherited from another subclass. Multilevel Inheritance ,the class A serves as a base class for the derived class B, which in turn serves as a base class for the derived class C. The class B is known as intermediate base class since it provides a link for the inheritance between A and C. Let us see an example of multilevel inheritance
package info.javaarch;
import java.util.List;
abstract class Vehicle { // Level 1; Parent Base class – Abstract class
public void vehicleType()
{
System.out.println(“Vehicle Type”);
}
public abstract void displayAllInfo(); // Abstract method
}
abstract class Car extends Vehicle{ // Level 2: Intermediate base class – Abstract
//…………..
// Implementation of Car
}
class BMW extends Car{ // Level 3: First concrete class in the inheritance tree.
@Override
public void displayAllInfo() { // Implementation of Abstract metho
// TODO Auto-generated method stub
}
}
Use multilevel inheritance and implement all the abstract method in the first concrete class. Write proper documentation for all the base class and implementation information to rule out any implementation issue in the subclass. Try to use “@Override” annotation to ensure we are overloading proper super class method.