Cohesion in Java
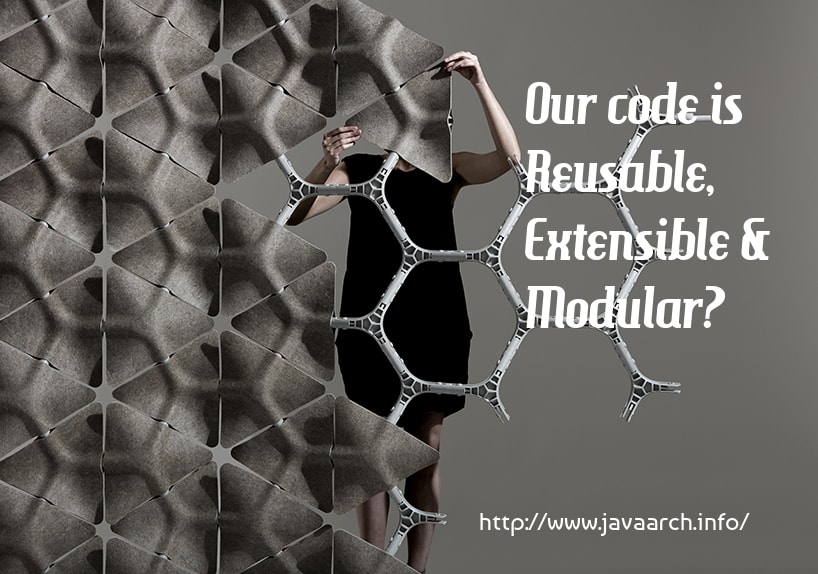
“Every software design document highlights that code should be reusable, extensible and robust. It measures the quality of the code. Everyone thinks their code has all the qualities. But we have seen many occurrences same code copied in more than one place. So our code not satisfies this first and foremost quality.
Let us discuss on the below code design.
Design 1: We have only one helper/worker at office. He does all the work. So we design only one worker class for the entire worker like Peon, security and gardener. Our class would look like,
class OfficeWorker{
void serveTea() { //serve tea to all the employees }
void checkBags() {//Check the visitors bags }
void waterThePlants() {// water the plants in garden.}
}
It works perfectly fine for the above use case and it would be sufficient to manage a single all in all office worker.Do you feel this would be fine or any issues you can find in this design? Alright. Let us say, tomorrow we hire one more worker only for gardening. Shall we use above class for that gardener. But mistakenly we call serveTea to the gardener, what would happen?. So we have to design another class Gardener and it will have only the waterThePlants() method.
class Gardener{
void waterThePlants() { // water the plants in garder.}
}
As of now it looks all problems solved. But what about code reusability. waterThePlants repeated in two different places. The same kind of code changes applicable if we hire security and Peon.
So while design the class, we have to check that Class does multiple, completely unrelated actions?. If answer is “Yes”, break the class into logical classes such as Gardener, Security and Peon. After split the OfficeWorker into 3 classes into three different classes as Gardener, Security and Peon. Every method is in it logically aligned to it class. Anyone can reuse our Gardener, Security and Peon. So no changes required in our code and completely reusable.
class Peon{ void serveTea() { //serve tea to all the employees } }
class Gardener{ void waterThePlants() {// water the plants in garden.} }
class Security{ void checkBags() {//Check the visitors bags } }
Design 2: We designed our classes. Finally the Security class would look like,
class Security{
void checkTheBag(){
code to open the bag;
code to check for explosive;
code to check for electronics;
code to close the bag and make entry in visitors diary;
}
}
Great, we have write the checkTheBag method to check the bag and make an entry in visitors diary. It would work as designed. Do you feel any change required here?
Let us say, company changes the rule that check only for electronics if the visitor is employee’s relative and not for weapons. But our method would check the weapon as well. Now we have to write another method to do all the open and close the bag. But only check for electronics. The open and close the bag, code to entry in visitors diary have to be copied in the newer method. Same code in 2 different places. then what is the point of code re-usability?
One more issue here is that the security would say to employee that he would check his relative’s bag. But the employee does not know what he checks in the bag only electronics or electronics and weapons both. Because method name is checkTheBag(). No clear explanation in that as company follows 2 different check for visitors and employee’s relatives. So no one knows what that method does.
To come out from this re-usability and understand-ability issue, the method should be split. Rewrite the method as below,
class Security{
void checkVisitorsBag(){
openTheBag();
checkElectronics();
checkExplosive();
closeTheBagandEntryinVisitor();
}
void checkEmployeeRelativesBag(){
openTheBag();
checkElectronics();
closeTheBagandEntryinVisitor ();
}
void openTheBag(){……}
void closeTheBagandEntryinVisitor (){…..}
void checkElectronics(){……..}
void checkExplosive(){….}
}
Now code is reused on security check. None of the code is duplicated and reused. Are you fine with the design now. The class is satisfies Reusable, Extensible and Modular qualities? If answer is “Yes”, you are wrong and continue reading .
Next if company says that government official’s visits office, just make an entry in visitor diary and no security check. Look at our code now. Entry in visitor diary code is in method closeTheBagandEntryinVisitor(). For government official’s no bag checking, so close the bag is invalid. We require only the entry in visitor code. We have to write new method only for entry in diary. Once again, the problem is code reusability. Same code of entry in visitor diary in two different places. You are wrong..!! Do not do that. Just design our classes in modular approach by writing a class to make entry in visitor diary “VisitorEntryMarker”. This class would make entry in visitor’s diary and would be used by other classes.
class Security{
void checkVisitorsBag(){
openTheBag();
checkElectronics();
checkExplosive();
closeTheBag ();
VisitorEntryMarker. entryinDiary();
}
void checkEmployeeRelativesBag(){
openTheBag();
checkElectronics();
closeTheBag ();
VisitorEntryMarker. entryinDiary();
}
void checkOfficialVisitors(){
VisitorEntryMarker. entryinDiary();
}
void openTheBag(){….}
void closeTheBag (){……}
void checkElectronics(){…..}
void checkExplosive(){….}
}
class VisitorEntryMarker(){
void entryinDiary(){// It would make entry in visitor diary.}
}
Now could you check again!! The above design is logically modelled and it is called as Highly Cohesive classes. Our code is Reusable, Extensible and Modular. Finally I would say “Yes”. If you have further refinement of this design, kindly update us by comments. We would discuss further on this design principle.
The same explained as cohesion in software design principles. You might have read as theory in your professional education. But here we discussed in more practical approach. In design principal, we call it as Highly Cohesive. In object oriented programming (OOPS), it is being emphasized as Data Encapsulation. Data Encapsulation is having the class variables and methods logically together in modular way. Cohesion helps us to design the class logically together. Whenever you explain the data encapsulation, use “Highly Cohesive” word as well. Interview tips shared:).
Key takeaways:
Class has variables and method which are designed logically and highly cohesive.
A Class or method should not perform multiple, completely unrelated actions.
A method should not do sequence of action even if are logically related. Split and call in sequence to enhance the reusability and understandability.
A method name should reflect it action. It enhances the readability of the class and methods.
Thanks for reading.!!
Learn more on Cohesion.